Overview
ProManage is a professional desktop application for companies that specialises in handling and executing projects. ProManage allow Manager and Employees of project teams to manage their team members and events easily. ProManage is optimized for those who prefer to work with a Command Line Interface (CLI) while still having the benefits of a Graphical User Interface (GUI).
This project portfolio documents my contributions to the development of the CS2113 project, as part of my team T16-2.
Summary of contributions
-
Major enhancement: added the ability to manage and view employees' events via the
invite
,remove
andselect
commands-
What it does: allows the user to invite employees to events, remove employees from events, and select and view an employee’s event schedule on the indicated date, month or year which is displayed on the event list panel.
-
Justification: This feature facilitates managing and scheduling of events among teams of employees who have different work schedules, and allow managers and employees to monitor and handle their event schedules easily. Schedule conflicts can be avoided and resolved easily with this enhancement.
-
Highlights: This feature considers whether the event an employee is invited to clashes with the particular employee’s work schedule, and guarantees that schedule conflict will not occur. The underlying
Event
model structure implemented for this enhancement is carefully designed and allows extension commandsviewmine
andselectEvent
to be easily implemented.
-
-
Minor enhancement:
-
set up the event list panel to display the events for the UI
-
added the
addEvent
command, which also warns the user when the planned event clashes or coincides with other existing events at the same location and time -
improved the
editEvent
command, which warns the user when the changed time or date of the edited event leads to schedule conflicts with any of the event’s attendees
-
-
Code contributed: [Functional and Test code]
-
Other contributions:
-
Project management:
-
Managed releases
v1.1
-v1.4
(6 releases) on GitHub -
Managed the issue tracker and project dashboard on GitHub
-
-
Enhancements to existing features:
-
Documentation:
-
Updated the User Guide to include
addEvent
,editEvent
,invite
,remove
andselect
command -
Updated the Developer Guide to include implementation details, use case, user stories and instructions for manual testing for
invite
andselect
command. -
Updated the
Model
,UI
andLogic
class diagram in Developer Guide
-
-
Community:
-
Tools:
-
Integrated GitHub Plugins (Coveralls, Travis CI, AppVeyor)
-
Automated team documentation build on GitHub pages
-
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Inviting employee to event: invite
Priority level: all
Invites an employee to an event.
Format: invite PERSON_INDEX to/EVENT_INDEX
Example:
-
invite 1 to/3
Invite 1st employee on employee list to 3rd event on event list
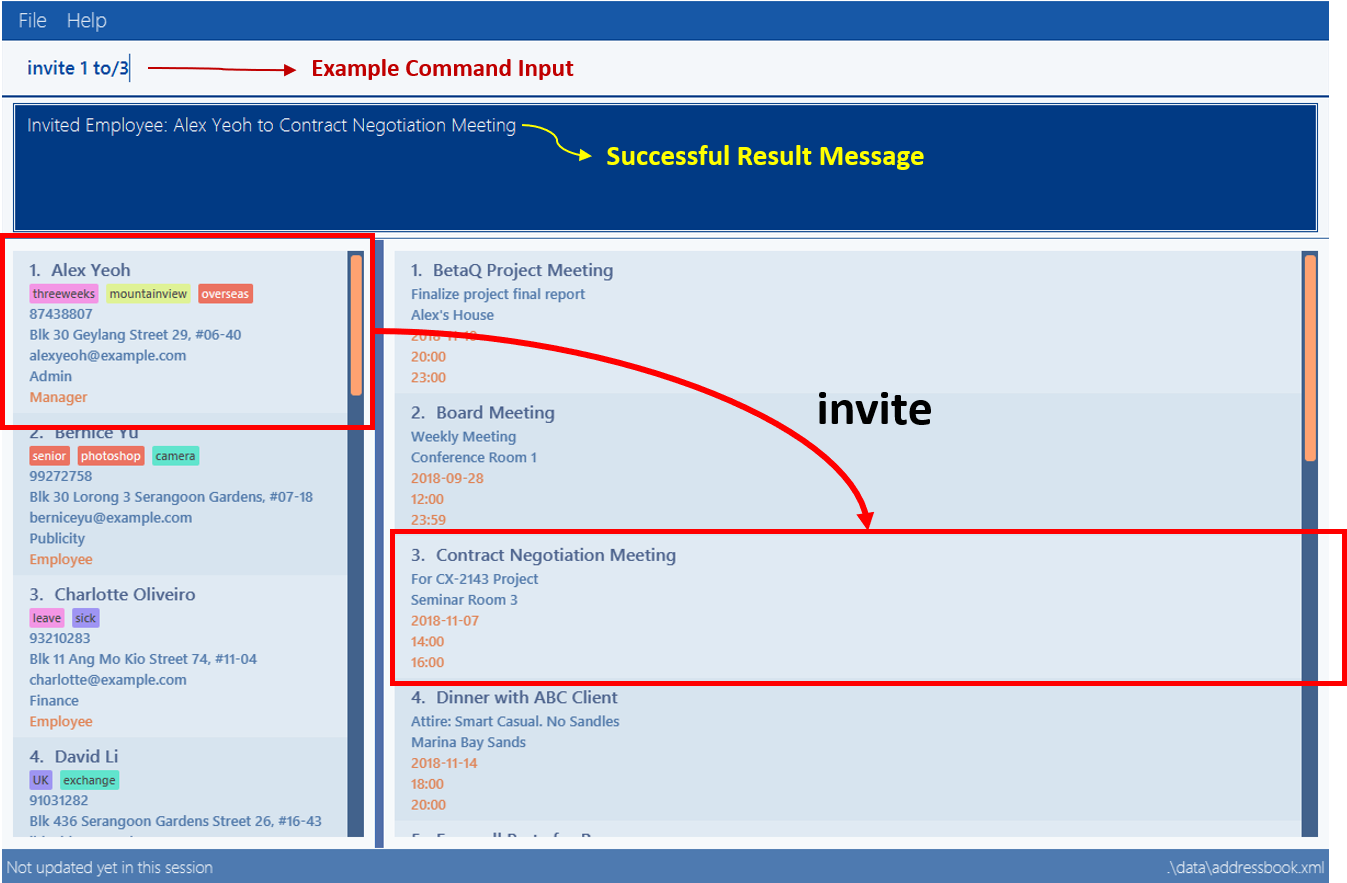
Removing employee from event: remove
Priority level: default
,manager
Removes an employee from the an event.
Format/Prompts: remove PERSON_INDEX from/EVENT_INDEX
Example:
-
remove 2 from/4
Remove 2nd employee at employee list from 4th event at event list
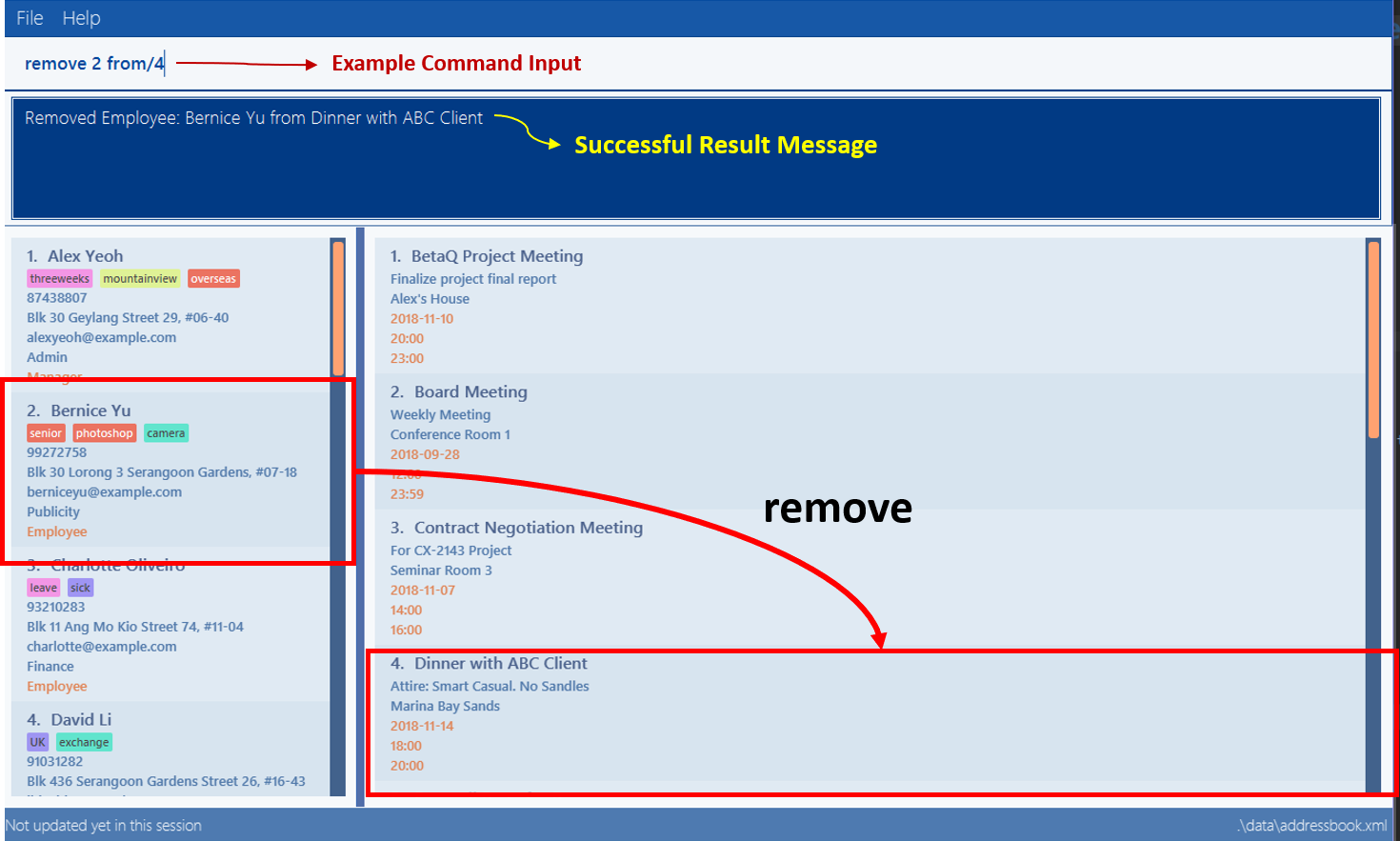
Selecting a person: select
Priority level: all
Selects an employee and view the selected employee’s events at the indicated time on the event list panel.
Format/Prompts:
Enter a command: select PERSON_INDEX [date/DATE] [m/MONTH] [y/YEAR]
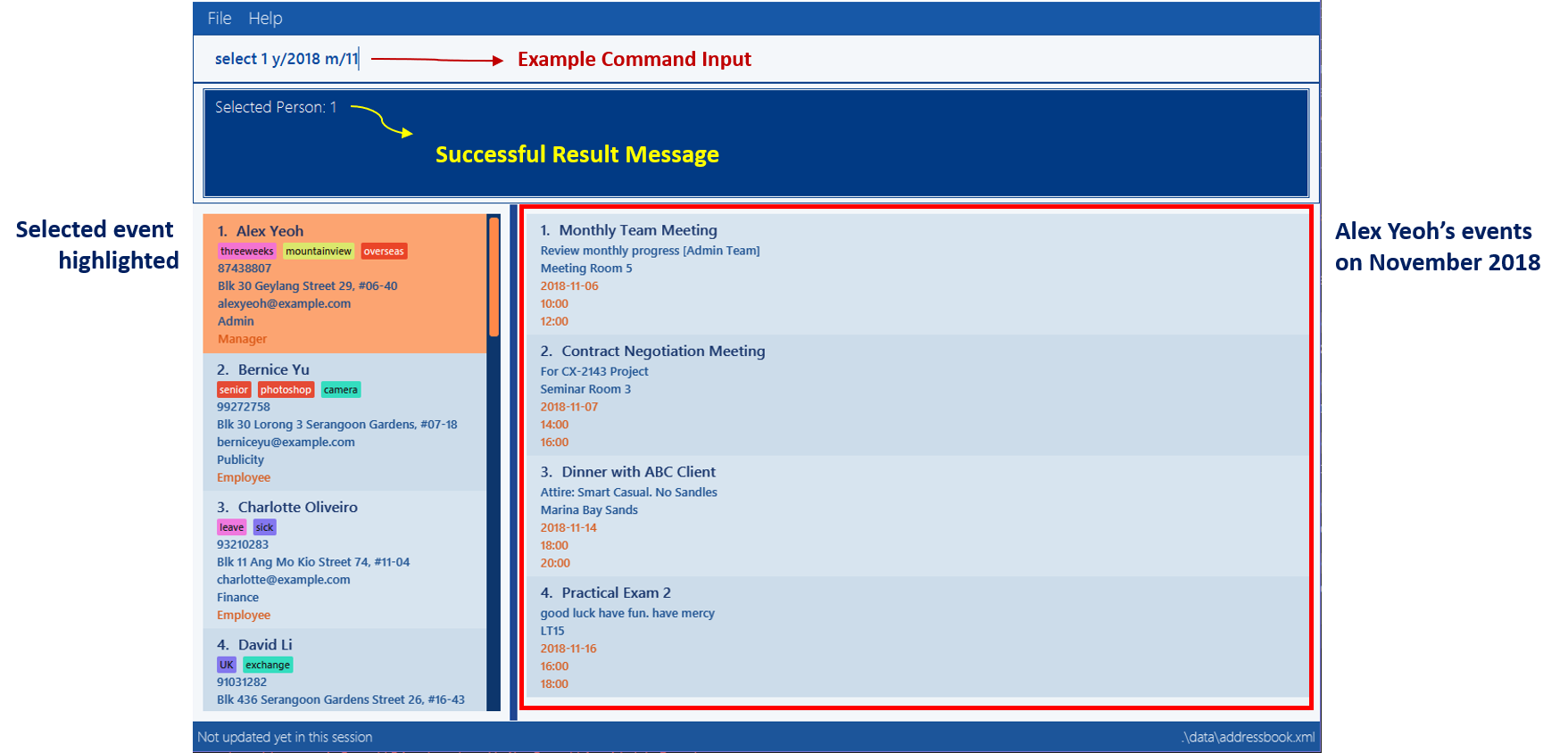
Examples:
-
select 1
: view all events of employee at index 1 -
select 1 date/2018-10-31
: view all events of employee at index 1 at 2018-10-31 -
select 1 m/08
: view all events of employee at index 1 in August -
select 1 y/2018
: view all events of employee at index 1 in 2018 -
select 1 y/2018 m/08
: view all events of employee at index 1 in August 2018
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Storage component
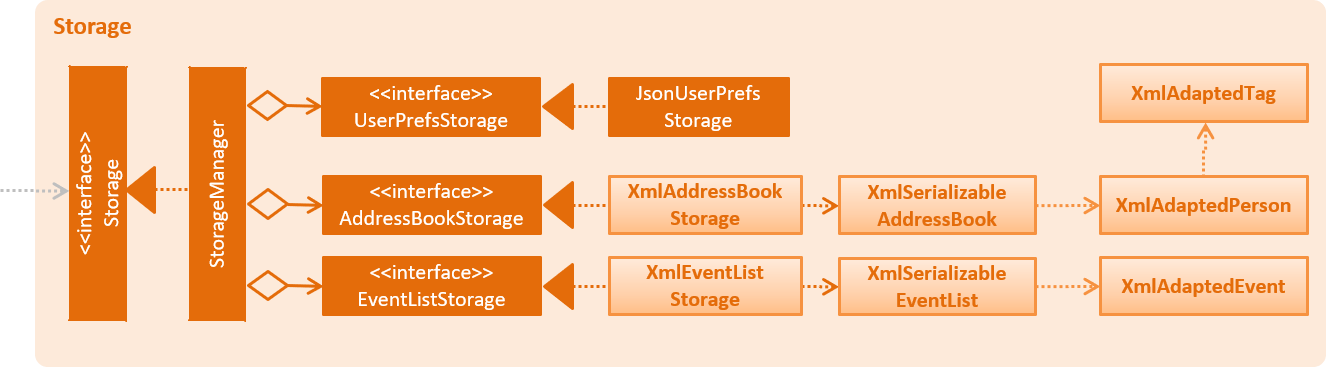
Model component
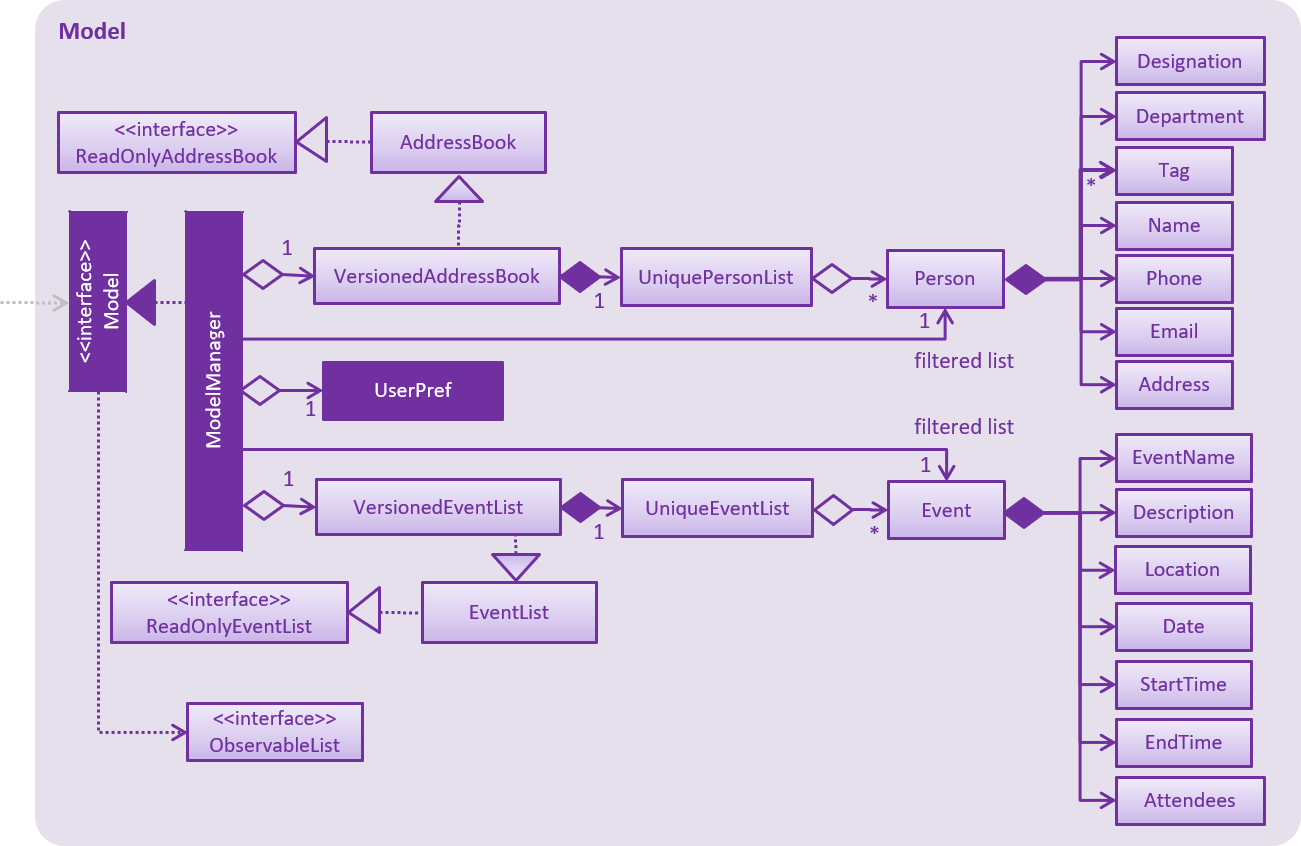
Invite feature
Current Implementation
The invite
command allows users to add attendees to an existing event, which is represented by an Event
object.
The command currently only allows users to add a single person to an event at a time, based on the indices on the UI.
This command adds the to-be-invited Person
's email, which is unique, to the chosen Event
, so that the attendees of a particular event is recorded.
The adding of attendees is facilitated by the class: Attendees
, which consists of a list of emails of type String
.
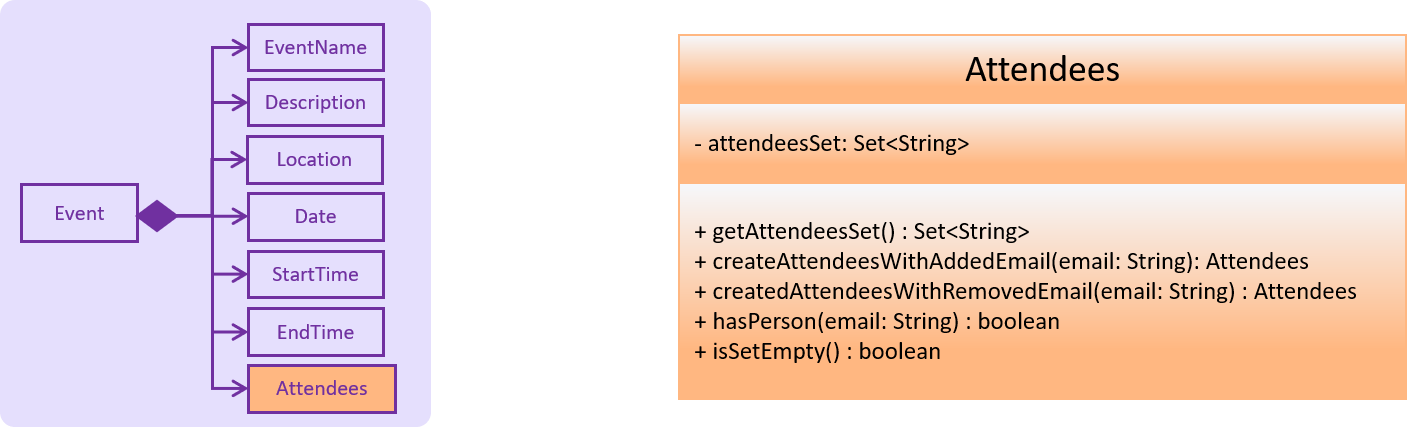
The following is a more detailed description of the Attendee
class:
-
Attendees
-
Each
Event
object consist of anAttendees
object, which is a list of the emails of the differentPerson
attending the event. -
This class is a wrapper class around the internal representation of a
Set
of emails. -
Only the email of the
Person
is recorded in anEvent
, as the email should be unique and uneditable.
-
Storing only the email of Person
in the Attendees
object of Event
saves memory storage and facilitates the select
, viewmine
and selectEvent
feature.
As we select a person, we can simply iterate through the event list and check whether the person’s email is in the attendees list.
Similarly, as we select an event, we can first obtain the list of emails from the Attendees
, and filter the employee list accordingly.
This command only executes successfully if the event does not not clash with the person’s schedule.
Given below is an illustration to describe the detection of whether two events clash, which is facilitated by the method hasClash
in Event
:
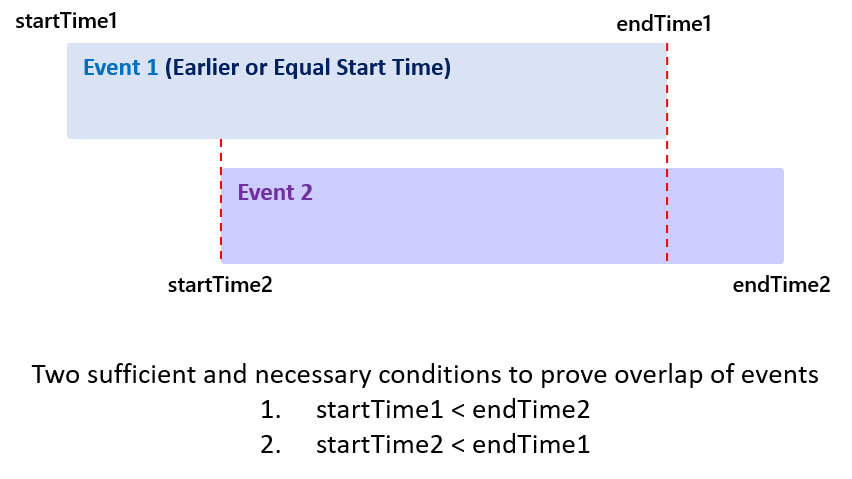
Event#hasClash
Thus, to check whether the event clashes with an employee’s schedule, we iterate through the ObservableList<Event>
and check whether the Event
1) contains the employee’s email in the Attendee
and 2) clashes with the selected event.
Implementation of RemoveCommand
is similar to InviteCommand
, but removes persons from Attendees
of events instead.
Execution of Command
Given below is an example usage scenario and how the invite mechanism works.
For example, the user inputs invite 1 to/1
to invite the 1st person in the address book to the 1st event in the event list.
Step 1. The command text is passed to an instance of the LogicManager
class.
Step 2. The LogicManager
instance calls ProManageParser#parseCommand
, which parses the invite command phrase.
Step 3. InviteCommandParser#parse
parses the person and event index. An instance of the InviteCommand
encapsulating the two indices information is then returned after parsing.
Step 4. Logic Manager
then executes this InviteCommand
by calling InviteCommand#execute
.
Step 5. The filtered person list and event list is first obtained by calling PersonModel#getFilteredPersonList
and EventModel#getFilteredEventList
. Based on the indices, the Person
and Event
is selected. Then, the Person
object’s email is obtained by calling Person#getEmail
Step 6. Two steps of verification is required before the person is invited to the event.
-
First,
Event#hasAttendee
checks whether the person is already invited to the event. -
Second,
Model#hasClash
checks whether the event clashes with the person’s schedule.
Step 7. After successful verification, the person’s email is added to the obtained Attendees
object. The new Attendees
object is then added to a new copy of the Event
object.
Step 8. The new Event
object is updated to the model by calling Model#updateEvent
.
Step 9. The InviteCommand#execute
finally returns a CommandResult
with a success message.
The sequence diagram below illustrates the execution of the invite
command.
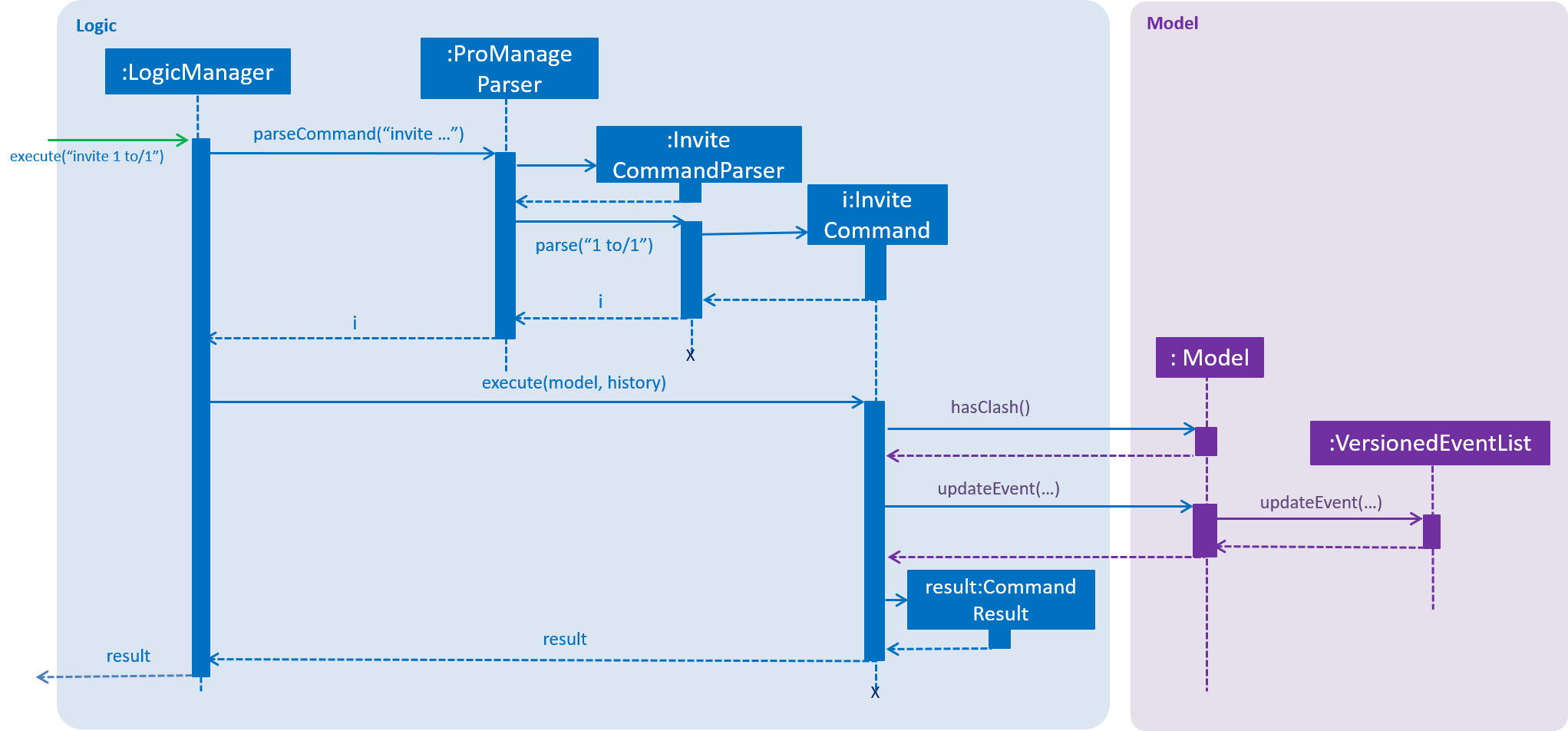
Design Consideration
Aspect: How to link Person
and Event
-
Alternative 1 (current choice): Saves only the emails of the Persons in the Attendees of Events.
-
Pros: Saves space. Able to filter EventList easily.
-
Cons: Requires going through the entire list of EventList to obtain details of events attended by the person.
-
-
Alternative 2: Saves the entire information of Events for each Persons.
-
Pros: Fast access to information of Events attended by any Persons.
-
Cons: Takes up a lot of storage space and memory. Many repeated items within the storage files.
-
Aspect: Data structure to support invite
command
-
Alternative 1 (current choice): Use
HashSet
to store persons emails.-
Pros: Easier and faster to retrieve or validate names stored. Able to handle duplicates easily.
-
Cons: Sequence of persons added is lost. Currently, this feature is not important for the project.
-
-
Alternative 2: Use
ArrayList
to store persons emails.-
Pros: Emails are stored in order of addition.
-
Cons: Inefficient when handling data.
-
Future Improvements
Adding entire department or multiple persons to an event
The current invite
command only invites one person to a chosen event based on the index chosen. This feature can be further extended to enable inviting more than one person or an entire department to an event.
One possible way to to implement this is to have the user
-
input a certain range (2-5) or
-
multiple indices (1,3,6,7,8) or
-
input the department name
The same concept can also be applied to events, being able to invite one person to multiple events with one single command.
Implementation of this additional feature would require changes to both InviteCommandParser
and InviteCommand#execute
. Additionally, handling of clashing events needs to be considered as it now involves more than one participant and event.
Similar improvements can be made to remove
command, being able to remove a person from a number of events with one single command.